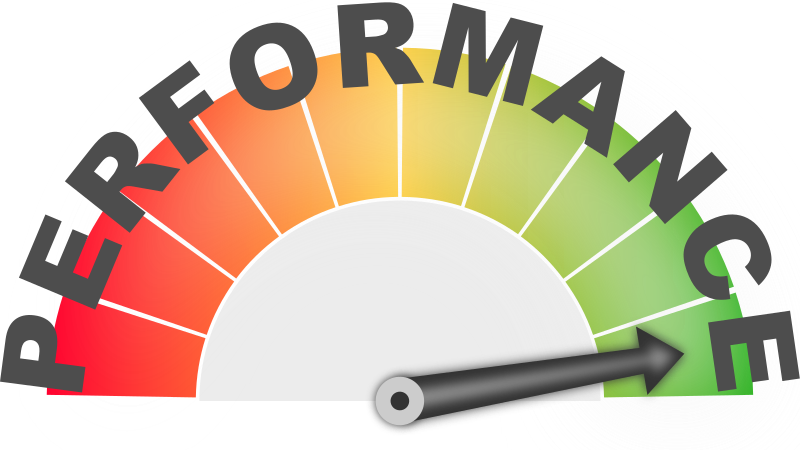
🧪 1. How do you measure and monitor performance in a .NET application?
📌 Why this is asked:
To check your practical experience with diagnosing and profiling performance issues.
✅ Answer:
I use tools like:
-
Application Insights or Elastic APM for real-time telemetry
-
dotTrace, PerfView, or Visual Studio Profiler for CPU/memory profiling
-
BenchmarkDotNet for micro-benchmarking methods
I monitor key metrics: CPU, memory usage, response time, database duration, and exceptions.
🗃️ 2. What’s the difference between IQueryable
and IEnumerable
? When would you use each?
📌 Why this is asked:
To assess understanding of deferred execution and query efficiency.
✅ Answer:
-
IQueryable
executes on the database side, translating LINQ into SQL — ideal for data filtering and pagination.
-
IEnumerable
brings all data into memory first, then filters in .NET.
I use IQueryable
for database operations and IEnumerable
for in-memory processing (e.g., business logic after query execution).
📉 3. How do you identify and fix slow SQL queries?
📌 Why this is asked:
To test how well you optimize database-heavy .NET applications.
✅ Answer:
I analyze the execution plan, index usage, and query stats using SQL Server Management Studio (SSMS). Common fixes include:
🧵 4. What are connection pooling and its performance impact?
📌 Why this is asked:
To verify database efficiency knowledge and resource management skills.
✅ Answer:
Connection pooling reuses existing DB connections instead of opening new ones, significantly improving performance. In .NET, it's enabled by default for SQL Server. I always ensure connections are closed properly (using
statement or await using
) to return them to the pool.
🧠 5. How do you reduce memory pressure in long-running .NET applications?
📌 Why this is asked:
To check your strategies for optimizing memory and avoiding leaks.
✅ Answer:
-
Reuse objects where possible (e.g., StringBuilder
, MemoryStream
)
-
Use value types or Span<T>
when applicable
-
Release unused references
-
Use ArrayPool<T>
or ObjectPool<T>
for frequent allocations
-
Profile heap usage and GC frequency with diagnostic tools
🔁 6. How do you implement caching in .NET to improve performance?
📌 Why this is asked:
To see how you reduce redundant operations and increase API speed.
✅ Answer:
I use:
-
In-memory cache (IMemoryCache
) for short-lived, frequent lookups
-
Distributed cache (e.g., Redis) for shared, scalable caching
-
Set expiration with sliding/absolute time
-
For EF Core, I cache expensive queries (e.g., read-heavy reports) and cache the DTOs, not EF entities
📦 7. What are EF Core tracking vs. no-tracking queries? How do they affect performance?
📌 Why this is asked:
To test knowledge of ORM internals and memory efficiency.
✅ Answer:
-
Tracking queries monitor entities for changes (used for updates)
-
No-tracking queries skip change tracking, reducing overhead — ideal for read-only operations
I use .AsNoTracking()
in read-heavy services to improve query speed and lower memory usage.
📍 8. How do you paginate data efficiently in SQL and .NET APIs?
📌 Why this is asked:
To assess your ability to handle large datasets in a performant way.
✅ Answer:
I use Skip()
and Take()
with proper OrderBy()
in LINQ:
dbContext.Customers
.OrderBy(c => c.Id)
.Skip((page - 1) * size)
.Take(size);
In SQL Server, I use OFFSET
and FETCH
. I avoid deep pagination (e.g., page 1000+) and offer cursor-based pagination if needed.
🔍 9. What’s the impact of lazy vs. eager loading in Entity Framework?
📌 Why this is asked:
To test your understanding of data access and performance trade-offs.
✅ Answer:
-
Lazy loading loads related data on demand, causing N+1 query issues if misused.
-
Eager loading (Include
) fetches related data in a single query, which is better for known use cases. I use eager loading for known navigations and disable lazy loading by default to avoid surprises.
🔄 10. How do you optimize loops and LINQ queries for performance?
📌 Why this is asked:
To test your ability to write high-performance code in .NET.
✅ Answer:
-
Minimize LINQ chains that enumerate multiple times
-
Use ToList()
only when necessary
-
Avoid closures and boxing in loops
-
Favor foreach
over for
for readability unless you need index
-
Profile lambda-heavy logic and refactor hot paths
I also use Span<T>
and array pooling in high-throughput scenarios.