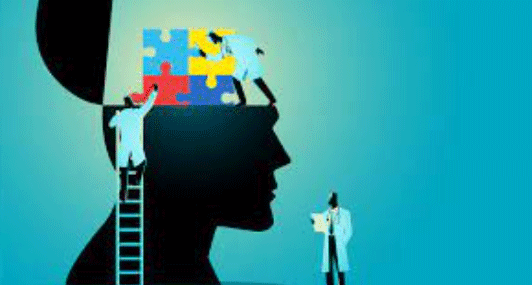
Conditional logic in C# is easy to write — but easy to get wrong when expressions grow more complex. I recently came across a test that combines boolean expressions, operator precedence, and DateTime
comparisons. It’s a great opportunity to sharpen your understanding of how C# evaluates logic at runtime.
Here’s the test:
public static void Main()
{
bool isForbidden = false;
bool isActive = true;
bool isDelayed = true;
DateTime threshold = DateTime.Now.AddDays(40);
if (!isForbidden && isActive && DateTime.Now > threshold || !isForbidden && DateTime.Now.AddDays(35) < threshold)
{
Console.WriteLine("Not forbidden");
}
if (!isForbidden && isActive && DateTime.Now > threshold || (isDelayed && !isActive || (DateTime.Now.AddDays(35) > threshold || isActive)))
{
Console.WriteLine("Either delayed or not");
}
if (!isForbidden && isActive && DateTime.Now < threshold && !isDelayed)
{
Console.WriteLine("Not delayed");
}
}
🔍 What Will Be Printed?
To evaluate this, let’s assume the code is running right now:
-
threshold = now + 40 days
-
DateTime.Now.AddDays(35)
is before threshold
-
DateTime.Now > threshold
is false
-
DateTime.Now < threshold
is true
✅ First Condition:
if (!isForbidden && isActive && DateTime.Now > threshold || !isForbidden && DateTime.Now.AddDays(35) < threshold)
🖨️ Output: Not forbidden
✅ Second Condition:
if (!isForbidden && isActive && DateTime.Now > threshold || (isDelayed && !isActive || (DateTime.Now.AddDays(35) > threshold || isActive)))
-
!isForbidden && isActive && DateTime.Now > threshold
→ false
-
isDelayed && !isActive
→ false
-
DateTime.Now.AddDays(35) > threshold
→ false
-
isActive
→ true
-
Final expression: false || (false || true)
→ true
🖨️ Output: Either delayed or not
❌ Third Condition:
if (!isForbidden && isActive && DateTime.Now < threshold && !isDelayed)
🖨️ Output: Nothing
✅ Final Console Output:
🎯 What You Should Learn From This
This code tests your understanding of complex boolean logic and how C# evaluates DateTime
conditions dynamically. Here are some important takeaways:
💡 Key Lessons
-
Operator Precedence
-
Short-Circuiting Behavior
-
With ||
, if the first condition is true, the rest is skipped
-
With &&
, if the first condition is false, the rest is skipped
-
DateTime Comparisons
-
DateTime.Now
changes every time it’s called
-
If you want consistency, store DateTime.Now
in a variable first
-
Write Readable Logic
👨💻 Final Thoughts
Even everyday conditions can become tricky when DateTime
and multiple booleans are combined. Use this kind of test to practice reading logic like a compiler — left to right, obeying precedence, and anticipating short-circuiting.
Have you come across similar logical puzzles or real-world condition bugs? Let’s chat in the comments