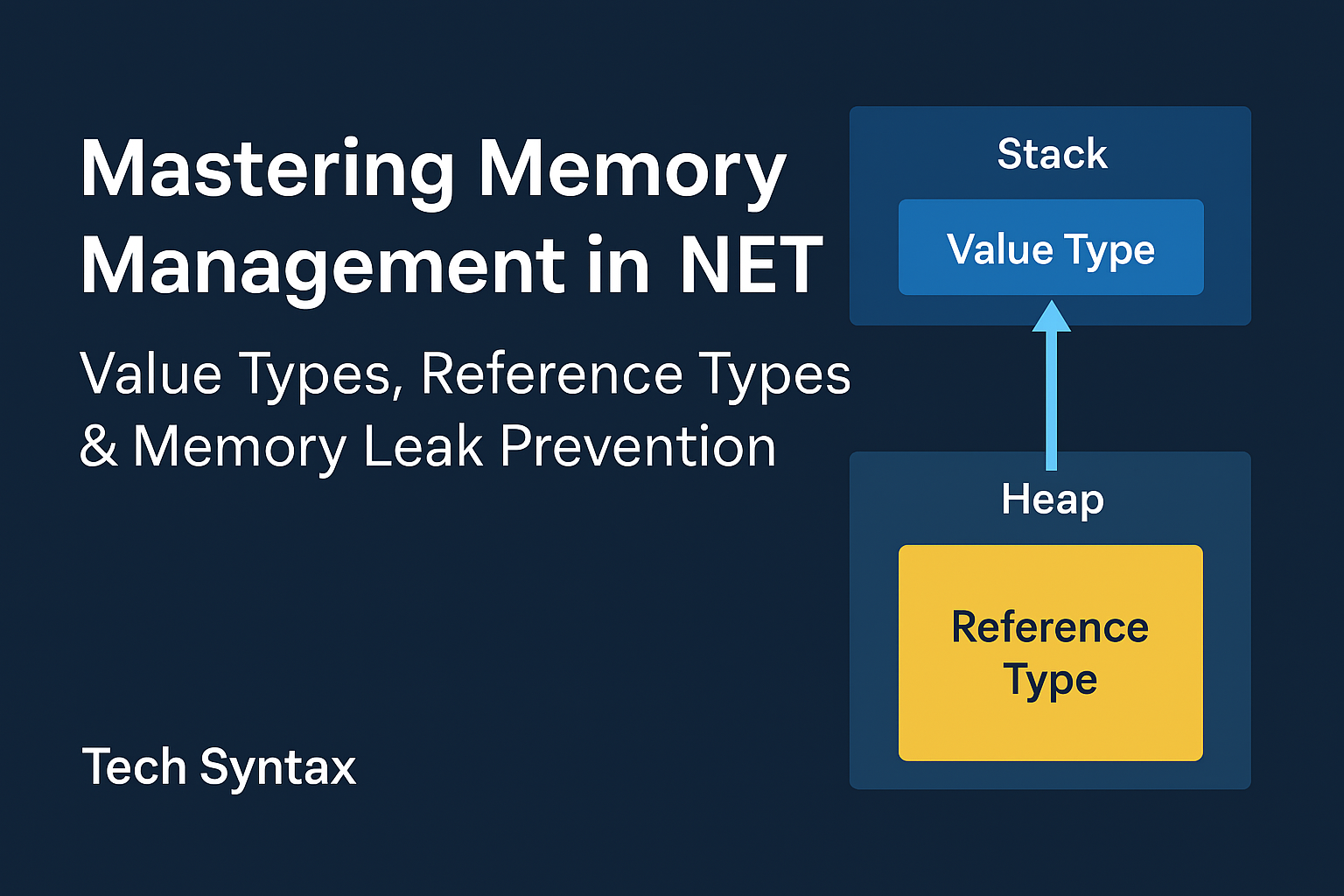
🧠 Memory management is one of the most critical concepts for building reliable, high-performance .NET applications — especially in financial and enterprise systems where performance and uptime matter.
In this post, we’ll break down how memory works in .NET, including:
-
The difference between value types and reference types
-
How memory is allocated and released
-
What causes memory leaks
-
Best practices to prevent memory issues
🔹 Stack vs Heap: The Foundation of .NET Memory Allocation
.NET uses two primary areas of memory:
-
Stack: Fast, organized, and stores short-lived variables
-
Heap: More flexible, stores objects that may live longer
✅ Value Types
Stored on the stack (unless part of an object)
int number = 42;
Value types are copied directly — which makes them fast and memory-efficient.
Examples: int
, float
, bool
, DateTime
, struct
✅ Reference Types
Stored on the heap, with a reference on the stack.
var user = new User();
The user
variable points to the object’s location in heap memory.
Examples: class
, string
, array
, object
, delegate
, interface
🔁 How .NET Releases Memory: The Garbage Collector
.NET's Garbage Collector (GC) automatically frees memory by removing unreachable objects on the heap.
It organizes memory in three generations:
-
Gen 0: Short-lived (e.g., method-local variables)
-
Gen 1: Medium-lived (e.g., temp business objects)
-
Gen 2: Long-lived (e.g., cached objects, singletons)
GC runs:
⚠️ When Do Memory Issues Happen in .NET?
Even with GC, managed applications can still leak memory or face performance issues due to poor coding practices.
Here are 5 common causes of memory issues in .NET:
1. Static References Holding Objects
Objects referenced by static
fields stay in memory for the lifetime of the app.
public static class Cache
{
public static LargeObject Cached = new LargeObject(); // stays forever
}
Fix: Avoid unnecessary static fields. Use cache expiration or weak references.
2. Event Handlers Not Unsubscribed
Subscribing to events but not unsubscribing prevents garbage collection.
service.Updated += myHandler; // memory leak
Fix: Always unsubscribe from events when the object is disposed or no longer needed.
3. Long-Lived Objects Holding Short-Lived Ones
For example, a singleton service stores HttpContext
or per-request data.
public static List<HttpContext> Requests = new(); // danger!
Fix: Don’t hold short-lived references in long-lived objects.
4. Large Object Heap (LOH) Fragmentation
Objects >85KB go into the LOH and aren't compacted often, causing fragmentation and bloat.
var buffer = new byte[100000]; // LOH
Fix: Use array pooling (ArrayPool<T>
) or streaming for large data.
5. Improper Use of IDisposable
Failing to dispose resources like SqlConnection
or StreamReader
causes leaks.
var conn = new SqlConnection(...); // not disposed
Fix: Always use using
blocks or call .Dispose()
properly.
using var conn = new SqlConnection(...);
🛡 Best Practices to Prevent Memory Issues
✅ Use using
or Dispose()
for any resource implementing IDisposable
✅ Unsubscribe from events
✅ Avoid long-living references to short-lived data
✅ Profile your app using dotMemory, Visual Studio Diagnostics, or PerfView
✅ Use WeakReference<T>
for caches
✅ Use async I/O and release large buffers.
🔎 Final Thoughts
Understanding memory allocation in .NET helps you write efficient, scalable, and bug-free code. It’s not just about preventing crashes — it’s about building software that performs well under pressure and survives in production.
If you're working on banking, fintech, or enterprise apps, memory management is something you should take seriously. A memory leak in a background service or web API can take hours — or days — to surface, and bring your system down when it matters most.