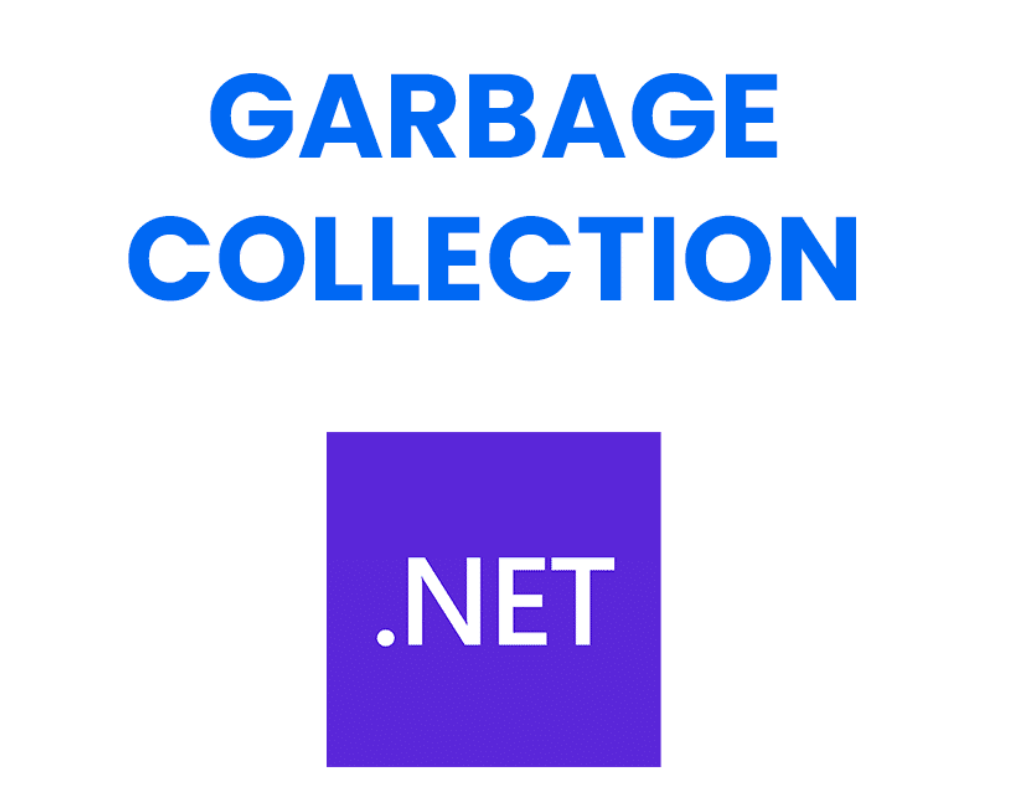
๐งน 1. What is Garbage Collection in .NET and how does it work?
๐ Why this is asked:
To verify that you understand how .NET handles automatic memory management behind the scenes.
โ
Answer:
Garbage Collection in .NET is a managed memory process that automatically reclaims memory occupied by objects no longer in use. The CLR tracks object references and periodically runs the GC to free up memory, improving performance and reducing memory leaks. The process is non-deterministic and uses generations (Gen 0, Gen 1, Gen 2) to optimize collection efficiency.
โป๏ธ 2. What are the different Garbage Collection generations in .NET?
๐ Why this is asked:
To test knowledge of .NET’s generational GC and memory optimization strategy.
โ
Answer:
.NET uses 3 GC generations:
-
Gen 0: Short-lived objects (collected most frequently)
-
Gen 1: Objects that survived Gen 0 (mid-lifetime)
-
Gen 2: Long-lived objects like caches or static data Generational GC improves efficiency by focusing on areas of memory where cleanup is more likely needed.
๐ 3. When does Garbage Collection occur in .NET?
๐ Why this is asked:
To check if you understand the triggers for GC and how to predict or influence it.
โ
Answer:
GC is triggered when:
-
The system is low on physical memory
-
Allocations exceed a threshold
-
GC.Collect()
is manually called (not recommended unless necessary) However, it’s non-deterministic, and you should never assume when GC will run unless explicitly forced.
๐งฐ 4. What is the difference between IDisposable
and a finalizer?
๐ Why this is asked:
To evaluate understanding of deterministic vs. non-deterministic cleanup.
โ
Answer:
-
IDisposable
is for deterministic cleanup using Dispose()
, typically used with using
blocks.
-
Finalizers (~ClassName()
) are non-deterministic and are called by the GC before reclaiming memory.
You should implement IDisposable
for resource management (e.g., file handles, DB connections), and only use finalizers for unmanaged resources.
โณ 5. What happens if you forget to call Dispose()
on an object?
๐ Why this is asked:
To test understanding of resource leaks and unmanaged memory risks.
โ
Answer:
If Dispose()
isn’t called, unmanaged resources like file handles or sockets may remain open, leading to memory/resource leaks. This is why using
statements or IAsyncDisposable
in async code are critical. In worst cases, it can exhaust system resources or crash services.
๐งฏ 6. How would you manage memory in a high-throughput .NET application?
๐ Why this is asked:
To assess your experience with performance tuning and avoiding memory pressure.
โ
Answer:
-
Reuse objects (object pooling) instead of frequent allocations
-
Avoid large object allocations when possible
-
Release references to unused objects
-
Minimize allocations in tight loops
-
Use Span<T>
, ArrayPool<T>
, and value types when appropriate
-
Profile memory usage with tools like dotMemory or PerfView
๐งช 7. What is a memory leak in .NET and how can you detect it?
๐ Why this is asked:
To check your ability to debug and resolve memory issues in long-running apps.
โ
Answer:
A memory leak occurs when objects are still referenced but no longer needed, preventing GC from reclaiming them. Common causes include static event handlers, caching without expiry, or long-lived objects holding references. You can detect leaks using memory profilers or by monitoring object growth with performance counters or diagnostics tools.
๐งต 8. What is the Large Object Heap (LOH) and how is it handled?
๐ Why this is asked:
To test your knowledge of memory segments and performance implications.
โ
Answer:
The Large Object Heap stores objects larger than 85,000 bytes. These are allocated directly in memory and are only collected during Gen 2 collections, making them more expensive to clean up. Fragmentation in the LOH can impact performance, so minimizing large allocations is advised. In .NET 4.5+, LOH compaction is optional via configuration.
๐ท 9. What tools do you use to analyze memory usage and GC performance?
๐ Why this is asked:
To verify real-world debugging and diagnostic experience.
โ
Answer:
I use:
-
dotMemory for in-depth memory analysis
-
Visual Studio Diagnostic Tools during debugging
-
PerfView and dotnet-counters for live monitoring
-
GC.GetTotalMemory() and GC.Collect()
during test cases These help identify memory leaks, GC overhead, and allocation patterns in live and test environments.
๐ 10. Should you ever manually call GC.Collect()
?
๐ Why this is asked:
To challenge understanding of GC control and performance trade-offs.
โ
Answer:
Generally, no. Forcing garbage collection interrupts normal execution and can degrade performance. It's only recommended in very specific scenarios like test automation, clearing temporary memory in short-lived apps, or after large resource releases when you're certain GC won’t run in time.