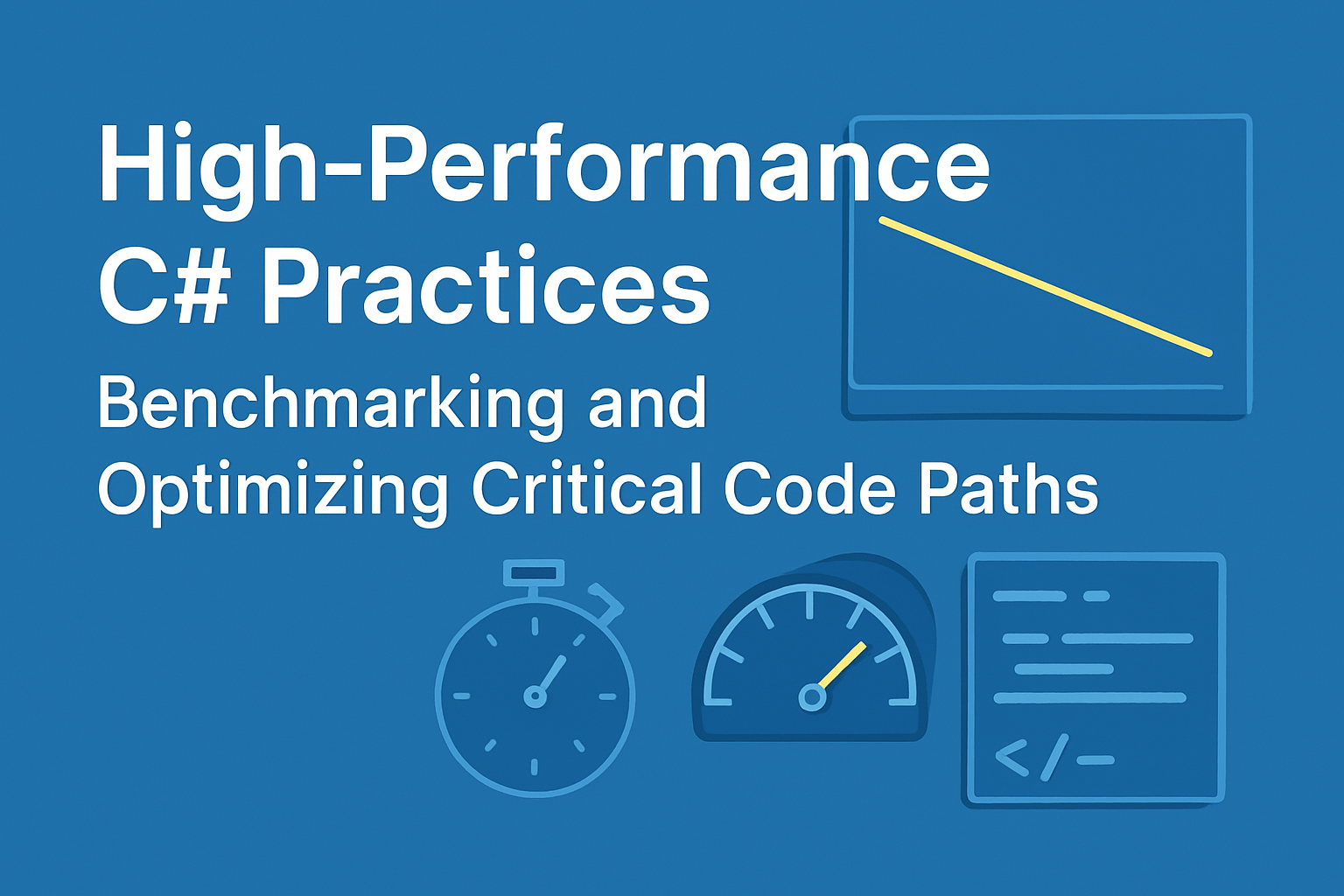
Why Performance Matters in C#
Performance is a key factor in building scalable and responsive applications. Whether you're developing high-traffic APIs, enterprise desktop apps, or real-time systems like trading engines or game backends, understanding and applying performance optimization techniques in C# can significantly improve your system's throughput, memory usage, and responsiveness. In this article, we explore essential performance comparison practices, when to use them, and how to measure their impact.
1. Value Types vs Reference Types
Understanding the memory model in .NET is foundational for writing performant code:
-
Value types (structs) are stored on the stack. They're fast to allocate and deallocate because the stack operates in a LIFO (last-in, first-out) manner.
-
Reference types (classes) are stored on the heap. They require memory allocation and deallocation by the garbage collector.
Why this matters: Using reference types for small, short-lived data (like coordinates, colors, or settings) adds overhead due to heap allocations and GC involvement.
Best Practice: Use value types for small, immutable data structures. Prefer struct
when size <16 bytes and it won’t be boxed or mutated by reference.
2. Boxing and Unboxing
Boxing happens when a value type is converted to object
, and unboxing when it's converted back. This results in additional memory allocations.
object boxed = 123; // boxing
int unboxed = (int)boxed; // unboxing
Why this matters: Repeated boxing in performance-critical paths (like within loops or LINQ queries) leads to memory pressure and CPU overhead.
Best Practice: Avoid boxing by using generics. For example, use List<int>
instead of ArrayList
or List<object>
.
3. String Operations
Strings are immutable in C#. Every modification creates a new string in memory.
Inefficient approach:
string result = "a" + "b" + "c"; // creates multiple intermediate strings
Efficient alternative:
var sb = new StringBuilder();
sb.Append("a");
sb.Append("b");
sb.Append("c");
Why this matters: In scenarios like report generation or log building, using +
repeatedly creates unnecessary memory allocations and slows performance.
Best Practice: Use StringBuilder
for concatenation inside loops or dynamic construction. Refer to our in-depth C# String Performance Showdown for benchmarks and deep dives.
4. Collections and Iteration
Choosing the right data structure and iteration method significantly impacts runtime performance:
-
List<T>
is preferred over ArrayList
for type safety and performance.
-
Dictionary<TKey, TValue>
is ideal for lookups, offering O(1) average-case complexity.
-
for
loops can be marginally faster than foreach
, especially with indexed collections.
Advanced Tip: For memory-critical scenarios (e.g., real-time processing), consider using Span<T>
and Memory<T>
to avoid heap allocations.
5. LINQ vs Loops
LINQ is elegant and expressive, but it can introduce performance overhead due to:
Example:
var activeUsers = users.Where(u => u.IsActive).ToList(); // concise but slower
vs
var activeUsers = new List<User>();
foreach (var u in users)
if (u.IsActive)
activeUsers.Add(u); // faster, especially in large collections
Best Practice: Use loops in hot paths or large data sets. Profile before replacing LINQ to preserve readability.
6. Async/Await Performance Tips
Async/await is powerful for improving responsiveness, especially for I/O-bound operations, but comes with overhead:
Best Practices:
-
Use ConfigureAwait(false)
to avoid context capture in libraries and background services.
-
Avoid async void
unless handling events.
-
Don’t wrap CPU-bound logic in Task.Run()
without profiling.
7. Avoiding Common Memory Pitfalls
Memory management plays a major role in C# performance:
-
Large Object Heap (LOH): Objects >85KB go to LOH and aren’t compacted regularly.
-
Excessive ToList()/ToArray(): Forces immediate enumeration and new allocations.
-
Memory Leaks: Holding onto references longer than needed (e.g., static lists, caches).
Best Practices:
-
Use pooling for frequently allocated objects (e.g., ArrayPool<T>
, ObjectPool<T>
).
-
Use lazy enumeration where possible to avoid upfront allocations.
-
Profile with tools like dotMemory or Visual Studio Diagnostics.
8. Benchmarking Best Practices
The only way to prove an optimization is to measure it. Tools like BenchmarkDotNet make it easy to write reproducible micro-benchmarks.
Example:
[MemoryDiagnoser]
public class StringTests {
[Benchmark]
public string ConcatWithPlus() {
string result = "";
for (int i = 0; i < 1000; i++)
result += "test";
return result;
}
}
Tips for Reliable Benchmarking:
-
Run benchmarks in Release mode.
-
Disable tiered JIT and background GC for accuracy.
-
Use MemoryDiagnoser
to spot allocations.
-
Always warm up before measuring.
9. Real-World Optimization Examples
Example 1: Replacing LINQ with foreach
in a loop reduced latency in a web API endpoint by 30% under load.
Example 2: Switching to StringBuilder
for composing large email bodies cut down memory usage by 60% and reduced GC pressure.
Example 3: Replacing nested List<T>
filtering with Dictionary<T, List<T>>
for faster lookups improved batch processing time by 90%.
Conclusion: Build for Readability First, Optimize with Purpose
Write code that is clean, correct, and maintainable—then profile and optimize based on actual performance needs. C# gives you powerful tools like Span<T>
, ValueTask
, BenchmarkDotNet
, and async/await. The key is knowing when and how to use them.
-
Don’t optimize prematurely.
-
Use benchmarks and diagnostics tools.
-
Apply best practices only when you have evidence they’re needed.
Ultimately, performance is about smart trade-offs. When you understand how C# manages memory, processes loops, and handles concurrency, you unlock the ability to build blazing-fast applications without sacrificing clean architecture.