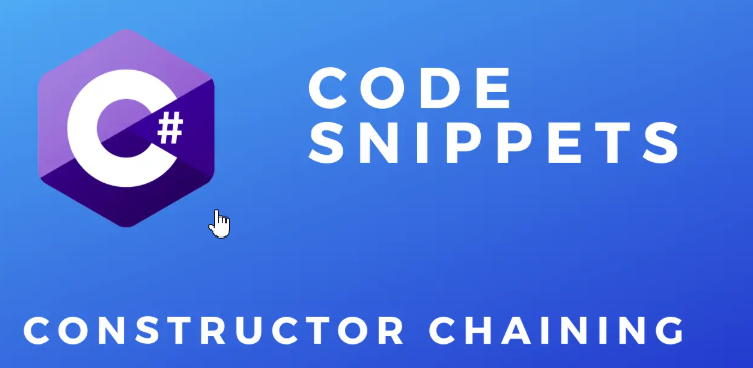
Constructors are often overlooked when we talk about object-oriented programming, but understanding how they interact — especially across base and derived classes — is critical. Here's a short but powerful challenge that explores constructor chaining and inheritance in C#.
Let’s analyze this snippet and predict the output.
🔍 The Challenge
private class Program
{
public static void Main()
{
ClassA refA = new ClassA();
ClassB refB = new ClassB();
}
}
class ClassA
{
public ClassA() : this(10)
{
Console.WriteLine("apple");
}
public ClassA(int pValue)
{
Console.WriteLine("banana");
}
}
class ClassB : ClassA
{
public ClassB() : base(0)
{
Console.WriteLine("tomato");
}
}
🧠 Step-by-Step Execution
1️⃣ First Line:
ClassA refA = new ClassA();
public ClassA() : this(10)
public ClassA(int pValue) { Console.WriteLine("banana"); }
Console.WriteLine("apple");
✅ So this line produces:
2️⃣ Second Line:
ClassB refB = new ClassB();
-
ClassB
is a derived class that explicitly calls:
-
Which invokes the same ClassA(int)
constructor that prints:
-
Then the body of ClassB
's constructor runs:
✅ So this line produces:
banana
tomato