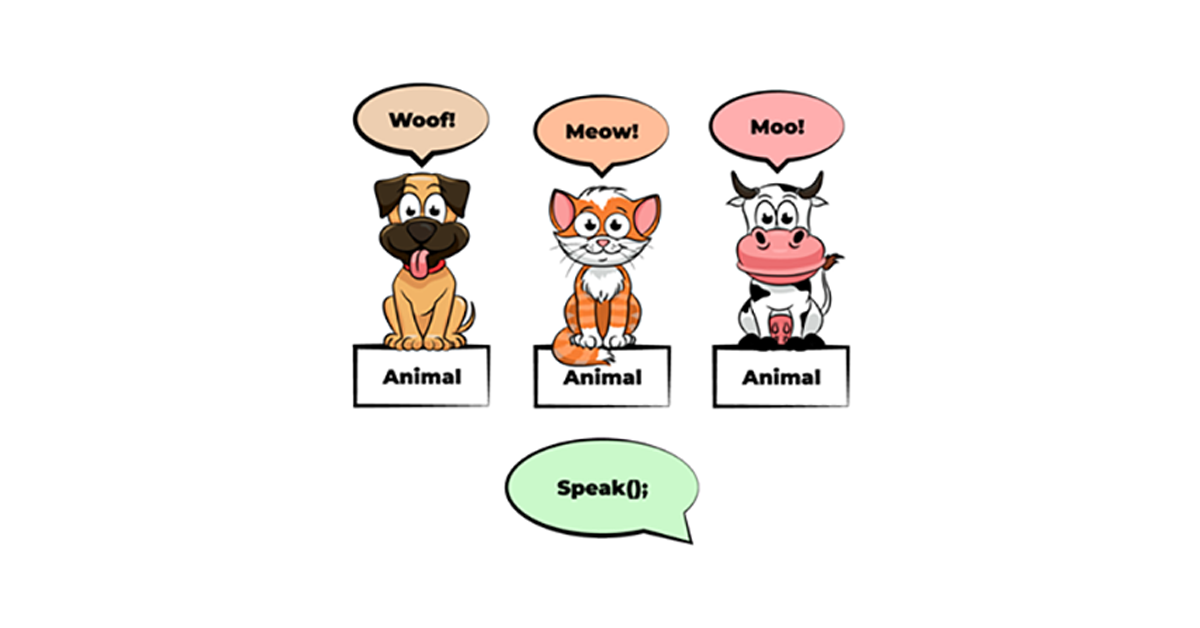
Polymorphism is one of the core principles of object-oriented programming — and C# gives you powerful tools to work with it. But there's a subtle trap many developers fall into when dealing with method hiding vs method overriding.
Let’s break down this C# challenge to understand what’s really happening and why the output might surprise you.
👇 The Challenge:
public class Animal // Base class (parent)
{
public virtual void animalSound()
{
Console.WriteLine("The animal makes a sound - #");
}
}
class Pig : Animal // Derived class (child)
{
public override void animalSound()
{
Console.WriteLine("The pig says: wee wee - #");
}
}
class Dog : Animal // Derived class (child)
{
public void animalSound() // method hiding, not overriding
{
Console.WriteLine("The dog says: bow wow - #");
}
}
class Program
{
public static void Main(string[] args)
{
Animal myAnimal = new Animal();
Animal myPig = new Pig();
Animal myDog = new Dog();
myAnimal.animalSound();
myPig.animalSound();
myDog.animalSound();
}
}
🔍 Predict the Output
Let’s go through it line by line:
1️⃣ myAnimal.animalSound();
The animal makes a sound - #
2️⃣ myPig.animalSound();
The pig says: wee wee - #
3️⃣ myDog.animalSound();
-
Object is of type Dog
, but declared as Animal
.
-
Dog
has a method named animalSound
, but it’s not overriding the base method.
-
So the base class method is called, because C# uses the reference type here.
-
✅ Output:
The animal makes a sound - #
✅ Final Output: