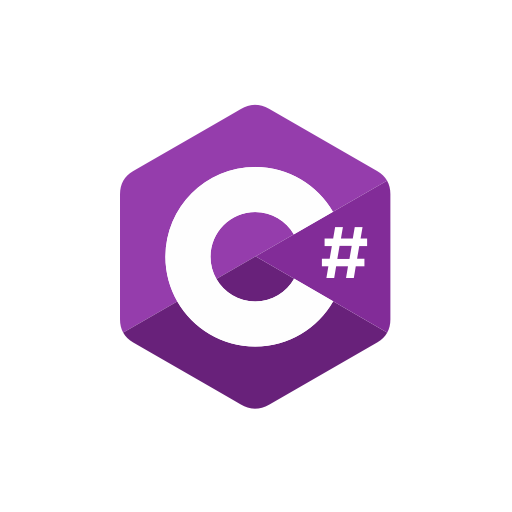
In a recent C# test, I came across this thought-provoking code snippet that’s deceptively simple — until you look a little closer.
public static void Main()
{
int sum = 0;
for (int i = 0; i < 10; i++)
{
if (i % 3 == 0)
{
DoIncrementStuff(ref i);
}
else
{
DoIncrementOtherStuff(i);
}
sum = sum + i;
}
// Console.WriteLine(sum);
}
public static void DoIncrementStuff(ref int a)
{
a++;
}
public static void DoIncrementOtherStuff(int a)
{
a++;
a++;
}
🧠 What Would Be Printed?
The question is: What would be the value of sum
when printed?
At first glance, it might look straightforward — but here’s where it gets interesting.
💡 Key Concepts Being Tested
This snippet tests your understanding of three important C# concepts:
-
Passing by Reference (ref
) vs. Passing by Value
-
How modifying the loop variable affects loop execution
-
The order of loop operations
🔎 Step-by-Step Breakdown
Iteration |
i (before) |
i%3==0? |
Method Call |
i (after call) |
Sum |
i (after loop increment) |
1 |
0 |
✅ Yes |
ref i → i++ = 1 |
1 |
1 |
2 |
2 |
2 |
❌ No |
by value → i remains 2 |
2 |
3 |
3 |
3 |
3 |
✅ Yes |
ref i → i++ = 4 |
4 |
7 |
5 |
4 |
5 |
❌ No |
by value → i remains 5 |
5 |
12 |
6 |
5 |
6 |
✅ Yes |
ref i → i++ = 7 |
7 |
19 |
8 |
6 |
8 |
❌ No |
by value → i remains 8 |
8 |
27 |
9 |
7 |
9 |
✅ Yes |
ref i → i++ = 10 |
10 |
37 |
loop ends |
✅ Final value of sum
: 37
🧪 Lessons You Can Take Away
-
Ref Parameters Are Powerful — and Dangerous
Using ref
can change control variables in loops. If not used carefully, this can lead to hard-to-find bugs.
-
Value Parameters Don't Affect the Caller
DoIncrementOtherStuff(i)
just plays with a local copy — no side effects on the original i
.
-
Loop Counter Manipulation Can Break Expectations
Modifying i
inside the loop body affects the flow in ways that might surprise you.
-
Be Mindful in Interviews
If you're in an interview setting, explaining why this code behaves the way it does will demonstrate your deep understanding — way beyond just giving the final output.
🧵 Conclusion
This short snippet is a goldmine for learning. It reinforces core concepts like parameter passing, loop behavior, and code predictability — all essential skills for a clean and bug-free C# development journey.
Have you seen similar questions in interviews? Or do you use ref
in any smart ways in your codebase? Let's discuss 👇