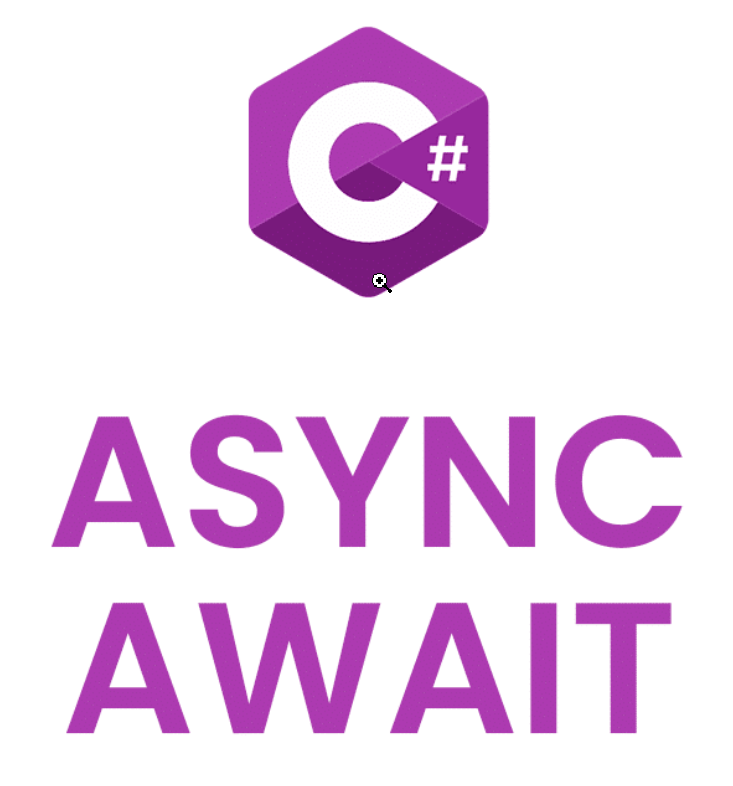
🔍 1. What’s the Difference Between async
and await
?
📌 Why this is asked:
To test your understanding of how asynchronous methods are declared and executed in C#.
✅ Answer:
The async
keyword enables a method to execute asynchronously, allowing it to return control early while it awaits operations. The await
keyword pauses the method until the awaited Task
completes. Behind the scenes, the compiler rewrites the method into a state machine that manages these transitions, simplifying asynchronous code compared to using callbacks or ContinueWith()
.
⚠️ 2. What Are Common Pitfalls of async/await
in .NET?
📌 Why this is asked:
To see if you're aware of the real-world issues that arise with misuse of async patterns.
✅ Answer:
-
Using async void
(except in event handlers), which prevents exception handling.
-
Blocking async code with .Result
or .Wait()
— leading to deadlocks.
-
Not propagating async all the way through the call chain.
-
Forgetting ConfigureAwait(false)
in reusable libraries.
-
Fire-and-forget tasks without exception handling or logging.
🔄 3. Why Should You Avoid .Result
or .Wait()
in Async Code?
📌 Why this is asked:
It checks whether you understand how deadlocks happen in synchronous waiting.
✅ Answer:
Using .Result
or .Wait()
blocks the calling thread. In ASP.NET, this can cause a deadlock since the thread is waiting for a result, while the result needs the thread to continue. The correct approach is always to use await
and keep the operation non-blocking.
⚙️ 4. When Should You Use ConfigureAwait(false)
?
📌 Why this is asked:
To test your understanding of synchronization context — especially in libraries and services.
✅ Answer:
Use ConfigureAwait(false)
when you don’t need to resume on the original synchronization context, such as in libraries, background services, or non-UI apps. This reduces overhead and improves performance by not capturing the context.
🧠 5. How Does async/await
Work Internally in .NET?
📌 Why this is asked:
Common in senior interviews to assess deeper knowledge of how the async model functions.
✅ Answer:
The compiler transforms the async
method into a state machine. Each await
creates a yield point, and the method returns a Task
. When the awaited task finishes, the continuation is resumed on the appropriate context. This keeps threads free and improves scalability.
🎯 6. When Should You Use Task
, ValueTask
, and void
in Async Methods?
📌 Why this is asked:
To test your knowledge of performance trade-offs and correct method signatures.
✅ Answer:
-
Use Task
for most async methods.
-
Use ValueTask
when the result may already be available and you want to avoid allocating a new Task.
-
Use async void
only for event handlers — never in business logic.
🧯 7. How Do You Handle Exceptions in Async Methods?
📌 Why this is asked:
To check if you understand exception flow in async and fire-and-forget scenarios.
✅ Answer:
Wrap your await
calls in try/catch
like synchronous code. For fire-and-forget tasks, use Task.Run
and catch exceptions inside the delegate:
_ = Task.Run(async () => {
try {
await DoWorkAsync();
} catch (Exception ex) {
_logger.LogError(ex, "Unhandled exception in background task");
}
});
⚡ 8. Can You Parallelize Async Operations? How?
📌 Why this is asked:
To evaluate your ability to optimize I/O-bound calls and improve app performance.
✅ Answer:
Yes. You can launch multiple tasks and await them together using Task.WhenAll()
:
var task1 = GetAAsync();
var task2 = GetBAsync();
await Task.WhenAll(task1, task2);
This runs tasks concurrently, reducing total execution time.
🧮 9. What’s the Difference Between CPU-Bound and I/O-Bound Async Work?
📌 Why this is asked:
To ensure you apply async programming only where it fits.
✅ Answer:
-
I/O-bound: External waiting (e.g., database, HTTP). Use async/await to avoid blocking threads.
-
CPU-bound: Heavy computation. Use Task.Run()
to run in the background thread pool.
🧩 10. How Would You Design a Service to Call 3 APIs Concurrently?
📌 Why this is asked:
A real-world question to test practical async usage and error handling.
✅ Answer: Call the APIs without awaiting immediately, then await them together:
var api1 = GetDataFromAAsync();
var api2 = GetDataFromBAsync();
var api3 = GetDataFromCAsync();
await Task.WhenAll(api1, api2, api3);
var combined = new {
A = await api1,
B = await api2,
C = await api3
};
This design boosts performance and ensures all responses are gathered before continuing.
✍️ Final Thoughts
Understanding and using async/await
effectively is critical for building scalable .NET applications. Whether you’re building banking APIs or handling concurrent service calls, mastering these concepts helps you write clean, efficient, and maintainable code.