A class should have one and only one reason to change, meaning that a class should have only one job.
How does this principle help us to build better software? Let’s see a few of its benefits:
- Testing — A class with one responsibility will have far fewer test cases
- Lower coupling — Less functionality in a single class will have fewer dependencies
- Organization — Smaller, well-organized classes are easier to search than monolithic ones
We have a Customer class that has more than one responsibility:
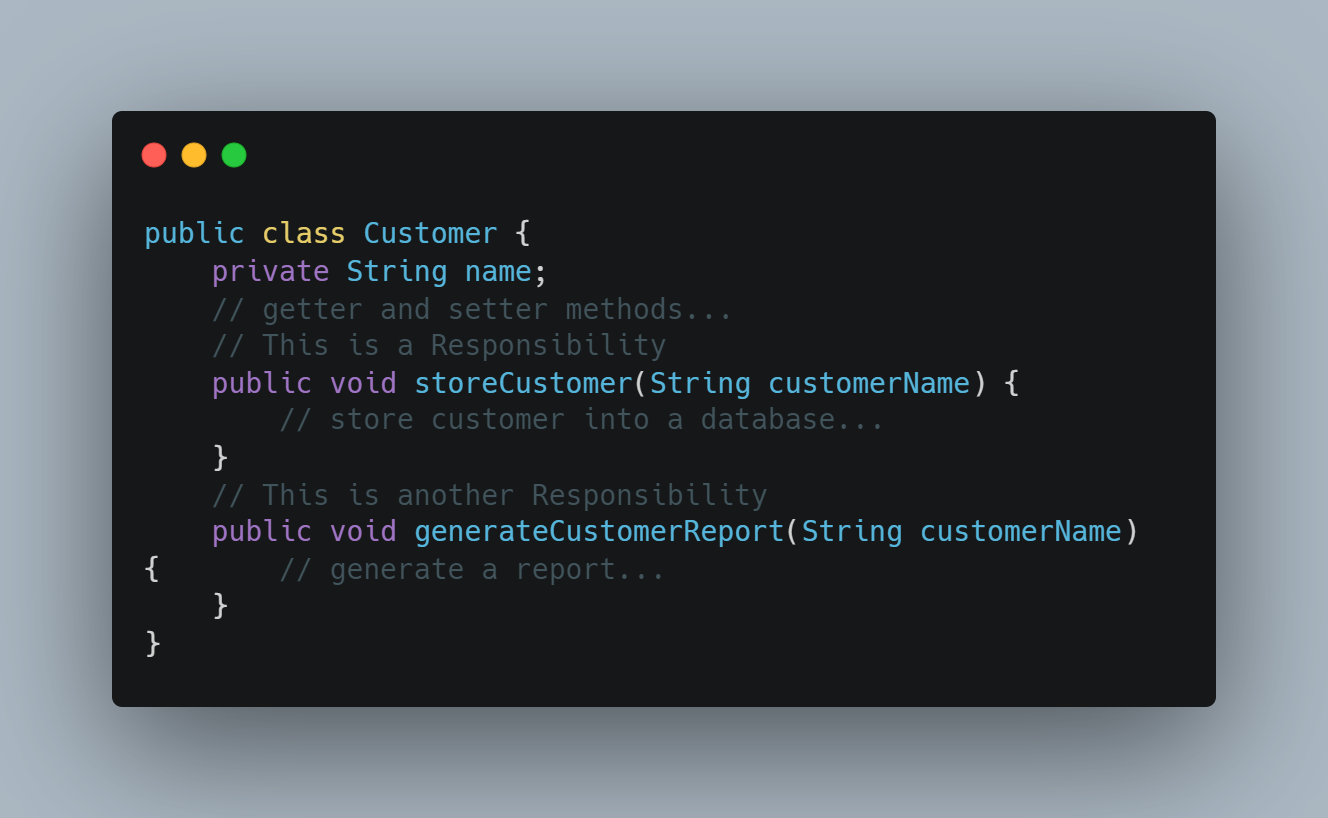
storeCustomer(String name) has the responsibility of storing a Customer into the database so it is a responsibility of persistence and should be out of the Customer class.
generateCustomerReport(String name) has the responsibility of generating a report about a Customer so it should also be out of the Customer class.
When a class has multiple responsibilities it is more difficult to understand, extend, and modify.
SRP solution:
We create different classes for each responsibility.
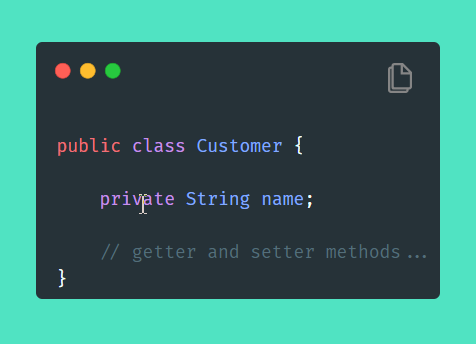
- CustomerDB class for the persistence responsibility:
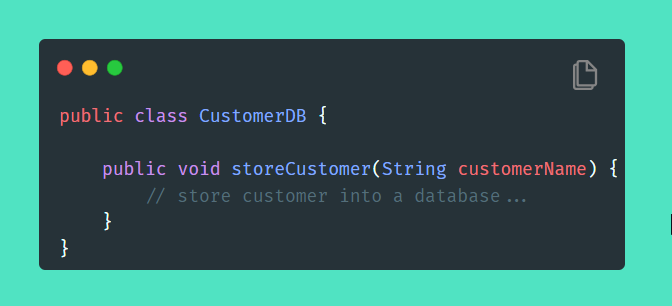
- CustomerReportGenerator class for the report generation responsibility:
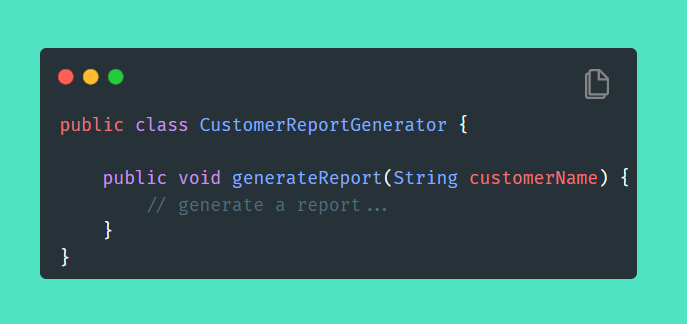
With this solution, we have some classes but each class with a single responsibility so we get a low coupling and to obtain better results.
Summary:
- The single responsibility principle is one of the most commonly used design principles in object-oriented programming. You can apply it to classes, software components, and microservices.
- To follow this principle, your class isn’t allowed to have more than one responsibility, e.g., the management of entities or the conversion of data types. This avoids any unnecessary, technical coupling between responsibilities and reduces the probability that you need to change your class. It also lowers the complexity of each change because it reduces the number of dependent classes that are affected by it. However, be reasonable.
- There is no need to have multiple classes that all hold just one function. Try to find the right balance when defining responsibilities and classes.